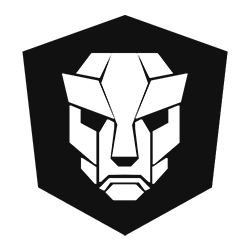
Spring Boot JSF ile Primefaces Kullanımı
Spring Boot JSF ile Primefaces Kullanımı
1 ) Spring Boot ile Jsf Uygulaması
Öncelikle Spring Boot ile JSF projesi nasıl oluşturulur anlatmıştık.Buradan o dersi inceleyip projenin son halini indirebilirsiniz.O proje üzerinde primefaces kütüphanesini ekleyeceğiz.
2 ) Primefaces Araçlarının Eklenmesi
Önceki derste anlattığımız hellojsf projesini açalım ve devam edelim.
2.1 ) Pom Xml Düzenlenmesi
Primefaces tema kütüphanesi maven repositoryde olmadığı için repo bilgisini ayrı ekledim
<!-- Primefaces Theme Repositories --> <repositories> <repository> <id>prime-repo</id> <name>Prime Repo</name> <url>http://repository.primefaces.org</url> </repository> </repositories>
Primefaces kütüphanesini ve repositorysini özel olarak eklediğimiz tema kütüphanesini de Dependency kısmına ekledim
<!-- Primefaces Dependency --> <dependency> <groupId>org.primefaces</groupId> <artifactId>primefaces</artifactId> <version>${primefaces.version}</version> </dependency> <dependency> <groupId>org.primefaces.themes</groupId> <artifactId>all-themes</artifactId> <version>${primefaces.theme.version}</version> </dependency>
Pom Xml son hali
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.atakancoban</groupId> <artifactId>helloJsf</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>helloJsf</name> <description>Spring Boot ile JSF Merhaba Dünya Örnegi!</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.3.RELEASE</version> <relativePath /> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> <jsf.version>2.2.9</jsf.version> <primefaces.version>6.1</primefaces.version> <primefaces.theme.version>1.0.10</primefaces.theme.version> </properties> <!-- Primefaces Theme Repositories --> <repositories> <repository> <id>prime-repo</id> <name>Prime Repo</name> <url>http://repository.primefaces.org</url> </repository> </repositories> <dependencies> <!-- Spring Dependency --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!-- JSF Dependency --> <dependency> <groupId>com.sun.faces</groupId> <artifactId>jsf-api</artifactId> <version>${jsf.version}</version> </dependency> <dependency> <groupId>com.sun.faces</groupId> <artifactId>jsf-impl</artifactId> <version>${jsf.version}</version> </dependency> <!-- Tomcat Embed Dependency --> <dependency> <groupId>org.apache.tomcat.embed</groupId> <artifactId>tomcat-embed-jasper</artifactId> </dependency> <!-- Primefaces Dependency --> <dependency> <groupId>org.primefaces</groupId> <artifactId>primefaces</artifactId> <version>${primefaces.version}</version> </dependency> <dependency> <groupId>org.primefaces.themes</groupId> <artifactId>all-themes</artifactId> <version>${primefaces.theme.version}</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
2.2 ) SpringBootApplication Sınıfı Düzenlenmesi
Spring uygulaması üzerinde jsf ayarlarını yaptığımız kısımda primefaces ile ilgili ayarlar ekliyoruz.Birer satır bilgiyi yorum satırı olarak ekledim detaylı araştırabilirsiniz.
@Bean public ServletContextInitializer servletContextInitializer() { return servletContext -> { servletContext.setInitParameter("com.sun.faces.forceLoadConfiguration", Boolean.TRUE.toString()); //Primefacesin ücretsiz temalarından bootstrap örneği yaptık değiştirebilirsiniz servletContext.setInitParameter("primefaces.THEME", "bootstrap"); //Primefaces client browser tarafında kontrol edilebilme örneğin textbox 10 karakter olmalı vs.. servletContext.setInitParameter("primefaces.CLIENT_SIDE_VALIDATION", Boolean.TRUE.toString()); //Xhtml sayfalarında commentlerin parse edilmemesi. servletContext.setInitParameter("javax.faces.FACELETS_SKIP_COMMENTS", Boolean.TRUE.toString()); //primefaces icon set için servletContext.setInitParameter("primefaces.FONT_AWESOME", Boolean.TRUE.toString()); }; }
Spring Boot Application(HelloJsfApplication.java) son hali
</pre> package com.atakancoban.helloJsf; import javax.faces.webapp.FacesServlet; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.web.servlet.ServletContextInitializer; import org.springframework.boot.web.servlet.ServletListenerRegistrationBean; import org.springframework.boot.web.servlet.ServletRegistrationBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import com.sun.faces.config.ConfigureListener; @SpringBootApplication @Configuration @ComponentScan("com.atakancoban") public class HelloJsfApplication { public static void main(String[] args) { SpringApplication.run(HelloJsfApplication.class, args); } //JSF Configration Başlangıc @Bean public ServletRegistrationBean<FacesServlet> facesServletRegistraiton() { ServletRegistrationBean<FacesServlet> registration = new ServletRegistrationBean<FacesServlet>(new FacesServlet(), new String[] { "*.xhtml" }); registration.setName("Faces Servlet"); registration.setLoadOnStartup(1); return registration; } @Bean public ServletContextInitializer servletContextInitializer() { return servletContext -> { servletContext.setInitParameter("com.sun.faces.forceLoadConfiguration", Boolean.TRUE.toString()); //Primefacesin ücretsiz temalarından bootstrap örneği yaptık değiştirebilirsiniz servletContext.setInitParameter("primefaces.THEME", "bootstrap"); //Primefaces client browser tarafında kontrol edilebilme örneğin textbox 10 karakter olmalı vs.. servletContext.setInitParameter("primefaces.CLIENT_SIDE_VALIDATION", Boolean.TRUE.toString()); //Xhtml sayfalarında commentlerin parse edilmemesi. servletContext.setInitParameter("javax.faces.FACELETS_SKIP_COMMENTS", Boolean.TRUE.toString()); //primefaces icon set için servletContext.setInitParameter("primefaces.FONT_AWESOME", Boolean.TRUE.toString()); }; } @Bean public ServletListenerRegistrationBean<ConfigureListener> jsfConfigureListener() { return new ServletListenerRegistrationBean<ConfigureListener>(new ConfigureListener()); } //JSF Configration Sonu } <pre>
2.3 ) Xhtml & Primefaces İlişkilendirmesi
Xhtml sayfasında primefaces araçlarını kullanmak için kütüphanesini eklememiz gerekli.
xmlns:p="http://primefaces.org/ui"
Xhtml sayfası
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" xmlns:f="http://java.sun.com/jsf/core" xmlns:h="http://java.sun.com/jsf/html" xmlns:p="http://primefaces.org/ui"> <h:head></h:head> <body> <h:form> <h:outputLabel value="#{helloBean.message}" /> <h:commandButton value="Hello World!" actionListener="#{helloBean.clickHelloWorldButton}" /> <p:outputLabel value="#{helloBean.message}"/> <p:commandButton value="Primefaces" icon="fa fa-fw fa-motorcycle " style="padding-bottom:5px" actionListener="#{helloBean.clickHelloWorldButton}"/> <p:calendar/> </h:form> </body> </html>
2.4 ) Önizleme
Primefaces araçları xml kısmı
<p:outputLabel value="#{helloBean.message}"/> <p:commandButton value="Primefaces" icon="fa fa-fw fa-motorcycle " style="padding-bottom:5px" actionListener="#{helloBean.clickHelloWorldButton}"/> <p:calendar/>
Burada icon= fa-motorcycle kısmı FONT_AWESOME eklentisi ile olmaktadır.
SpringApplication ayarları kısmında bunu eklemiştik.Detayları Burada.
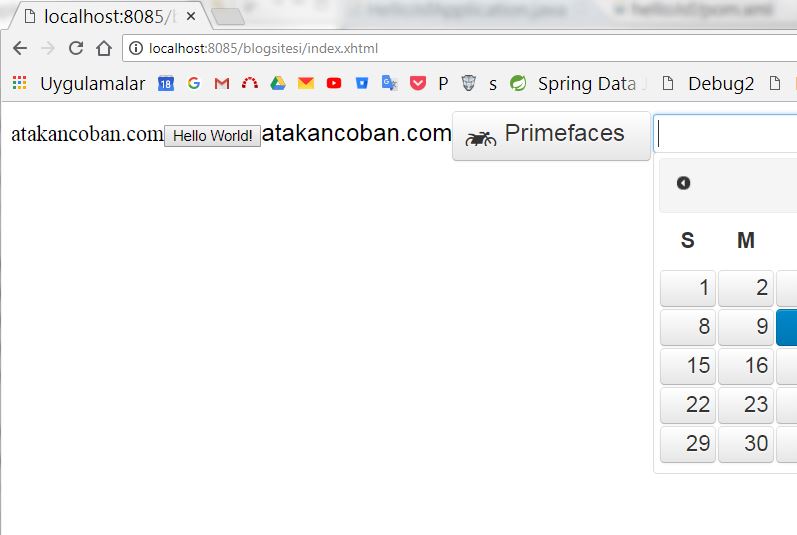
Projeyi Buradan indirebilirsiniz
Benzer Bloglar : Şükrü Çakmak